Infinite Scrolling Logos Animation using Tailwind and Framer Motion
Introduction
This tutorial will guide you through the process of creating an infinite-scrolling logo animation using Tailwind and Framer Motion. We'll cover the basics of setting up the project, creating the animation, and styling the elements.
Prerequisites
Before we begin, make sure you have the following installed:
- React.js or (Any other react framework)
- Tailwind CSS
- Framer Motion
Setting Up the Project
To get started, create a new Next.js project using the following command:
npx create-next-app@latest infinite-scolling-logos-animation
Next, navigate to the project directory and install the necessary dependencies:
cd infinite-scolling-logos-animation
npm install tailwindcss framer-motion
Infinite-Scrolling Logos Animation
Our version of the infinite scrolling logos animation, which we'll call the "Infinite-Scrolling Logos Animation," adds some stylish twists:
- Continuous loop of scrolling logos
- Smooth fade-in and fade-out at the edges
Here's what we're aiming for:
Trusted by the world's most innovative companies
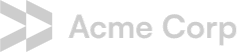
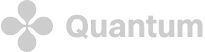

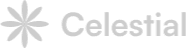
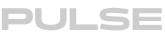
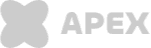
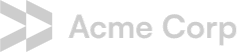
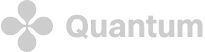

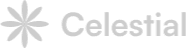
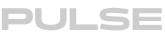
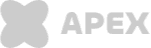
Implementation Steps
Step 1: Create the Infinite-Scrolling Logos Animation Component
First, let's set up our Infinite-Scrolling-Logos-Animation.tsx
component:
import React from 'react';
const InfiniteScrollingLogosAnimation = () => {
return (
<div>Infinite-Scrolling Logos Animation Component</div>
);
};
export default InfiniteScrollingLogosAnimation;
Step 2: Import Logos from Public Folder and Create Array of Logos
We'll use an array of logos for our scrolling content:
import React from 'react';
mport acmeLogo from '@/public/logos/acme.png';
import quantumLogo from '@/public/logos/quantum.png';
import echoLogo from '@/public/logos/echo.png';
import celestialLogo from '@/public/logos/celestial.png';
import pulseLogo from '@/public/logos/pulse.png';
import apexLogo from '@/public/logos/apex.png';
const CompanyLogoData: Array<{ src: any; alt: string }> = [
{ src: acmeLogo, alt: 'Acme Logo' },
{ src: quantumLogo, alt: 'Quantum Logo' },
{ src: echoLogo, alt: 'Echo Logo' },
{ src: celestialLogo, alt: 'Celestial Logo' },
{ src: pulseLogo, alt: 'Pulse Logo' },
{ src: apexLogo, alt: 'Apex Logo' },
];
const InfiniteScrollingLogosAnimation = () => {
return (
<div>Infinite-Scrolling Logos Animation Component</div>
);
};
export default InfiniteScrollingLogosAnimation;
Step 3: Implement the Infinite-Scrolling Logos Animation
Now, let's build out our component with the necessary HTML structure and CSS classes:
import { DotIcon } from '@radix-ui/react-icons';
import React from 'react';
import acmeLogo from '@/public/logos/acme.png';
import quantumLogo from '@/public/logos/quantum.png';
import echoLogo from '@/public/logos/echo.png';
import celestialLogo from '@/public/logos/celestial.png';
import pulseLogo from '@/public/logos/pulse.png';
import apexLogo from '@/public/logos/apex.png';
const CompanyLogoData: Array<{ src: any; alt: string }> = [
{ src: acmeLogo, alt: 'Acme Logo' },
{ src: quantumLogo, alt: 'Quantum Logo' },
{ src: echoLogo, alt: 'Echo Logo' },
{ src: celestialLogo, alt: 'Celestial Logo' },
{ src: pulseLogo, alt: 'Pulse Logo' },
{ src: apexLogo, alt: 'Apex Logo' },
];
const InfiniteScrollingLogosAnimation = () => {
return (
<div className="container p-5">
<h2 className="text-center text-xl text-white/70 my-5">
Trusted by the world's most innovative companies
</h2>
<div className="flex relative overflow-hidden before:absolute before:left-0 before:top-0 before:z-10 before:h-full before:w-10 before:bg-gradient-to-r before:from-zinc-950 before:to-transparent before:content-[''] after:absolute after:right-0 after:top-0 after:h-full after:w-10 after:bg-gradient-to-l after:from-zinc-950 after:to-transparent after:content-['']">
<motion.div
transition={{
duration: 10,
ease: 'linear',
repeat: Infinity,
}}
initial={{ translateX: 0 }}
animate={{ translateX: '-50%' }}
className="flex flex-none gap-16 pr-16"
>
{[...new Array(2)].fill(0).map((_, index) => (
<React.Fragment key={index}>
{CompanyLogoData.map(({ src, alt }) => (
<Image
key={alt}
src={src}
alt={alt}
className="h-8 w-auto flex-none"
/>
))}
</React.Fragment>
))}
</motion.div>
</div>
</div>
)};
export default InfiniteScrollingLogosAnimation;
Step 4: Use the Infinite-Scrolling Logos Animation Component
Finally, import and use the Infinite-Scrolling-Logos-Animation
component in your desired page or component:
import InfiniteScrollingLogosAnimation from '@/components/Infinite-Scrolling-Logos-Animation';
export default function Home() {
return (
<div>
<h1>Welcome to My App</h1>
<InfiniteScrollingLogosAnimation />
</div>
);
}
Conclusion
You've now created a stylish Infinite-Scrolling Logos Animation using React and CSS animations. This effect can be customized further by adjusting colors, speeds, and content to fit your specific design needs.
Further Enhancements
- Make the component more dynamic by passing the logos as props
- Implement pause on hover functionality for better user interaction
Remember, while eye-catching animations can enhance user experience, they should be used judiciously to avoid overwhelming your users.